Introduction:
As a SQL Server DBA or SQL Developer, the performance and stability of your MSSQL server is of utmost importance. One of the key factors in achieving this is proper query optimization. Inefficient queries can lead to slow response times, poor performance, and even system crashes. However, by utilizing advanced techniques and best practices, you can optimize your queries and improve the overall performance of your MSSQL server. In this post, we will explore 15 advanced tips for MSSQL Server query optimization, complete with examples of inefficient and optimized queries under each tip.
15 advanced tips for MSSQL Server query optimization:
Tip 1: Use proper indexing to improve query performance.
Inefficient query:
SELECT * FROM customers WHERE last_name = ‘Smith’ |
Optimized query:
SELECT * FROM customers WITH (INDEX(idx_last_name)) WHERE last_name = ‘Smith’ |
Tip 2: Avoid using the “SELECT *” statement, as it can slow down query performance. Instead, only select the specific columns needed for the query.
Inefficient query:
SELECT * FROM orders |
Optimized query:
SELECT order_id, order_date, customer_id FROM orders |
Tip 3: Use the EXPLAIN or EXPLAIN ANALYZE command to see the execution plan of a query and identify any potential bottlenecks.
Inefficient query:
SELECT * FROM orders WHERE customer_id = ‘123’ |
Optimized query:
EXPLAIN ANALYZE SELECT * FROM orders WHERE customer_id = ‘123’ |
Tip 4: Use subqueries and joins sparingly, as they can slow down query performance. Instead, try to use a single, well-optimized query.
Inefficient query:
SELECT * FROM orders o WHERE o.customer_id = (SELECT c.customer_id FROM customers c WHERE c.last_name = ‘Smith’) |
Optimized query:
SELECT * FROM orders o INNER JOIN customers c ON o.customer_id = c.customer_id WHERE c.last_name = ‘Smith’ |
Tip 5: Use the LIMIT and OFFSET clauses to limit the number of rows returned by a query, instead of using the TOP clause.
Inefficient query:
SELECT TOP 10 * FROM orders |
Optimized query:
SELECT * FROM orders LIMIT 10 OFFSET 0 |
Tip 6: Use the UNION, UNION ALL, INTERSECT and EXCEPT operators to combine multiple queries into a single query.
Inefficient query:
SELECT * FROM orders WHERE customer_id = ‘123’UNIONSELECT * FROM orders WHERE customer_id = ‘456’ |
Optimized query:
SELECT * FROM orders WHERE customer_id IN (‘123′,’456’) |
Tip 7: Use the GROUP BY and HAVING clauses to group and filter query results, instead of using the WHERE clause.
Inefficient query:
SELECT * FROM orders WHERE order_date >= ‘2022-01-01’ AND order_date <= ‘2022-12-31’ |
Optimized query:
SELECT order_date, COUNT(*) FROM orders GROUP BY order_date |
Tip 8: Use the INNER JOIN, LEFT JOIN, RIGHT JOIN and FULL OUTER JOIN clauses to join tables, instead of using subqueries or multiple SELECT statements.
Inefficient query:
SELECT * FROM orders o WHERE o.customer_id = (SELECT c.customer_id FROM customers c WHERE c.last_name = ‘Smith’) |
Optimized query:
SELECT * FROM orders o INNER JOIN customers c ON o.customer_id = c.customer_id WHERE c.last_name = ‘Smith’ |
Tip 9: Use the CASE statement to perform conditional logic in queries, instead of using multiple OR or AND clauses.
Inefficient query:
SELECT * FROM orders WHERE (status = ‘completed’ OR status = ‘shipped’) AND customer_id = ‘123’ |
Optimized query:
SELECT * FROM orders WHERE CASE WHEN status = ‘completed’ THEN 1 WHEN status = ‘shipped’ THEN 1 ELSE 0END = 1 AND customer_id = ‘123’ |
Tip 10: Use the COUNT, SUM, AVG, MIN and MAX aggregate functions to summarize query results, instead of using the GROUP BY clause.
Inefficient query:
SELECT order_id, SUM(price) FROM order_items GROUP BY order_id |
Optimized query:
SELECT order_id, SUM(price) FROM order_items GROUP BY order_id |
Tip 11: Use the LIKE and NOT LIKE operators to search for specific patterns in data, instead of using the = and != operators.
Inefficient query:
SELECT * FROM customers WHERE last_name = ‘Smi%’ |
Optimized query:
SELECT * FROM customers WHERE last_name LIKE ‘Smi%’ |
Tip 12: Use the DISTINCT keyword to remove duplicate rows from query results, instead of using the GROUP BY clause.
Inefficient query:
SELECT order_id, customer_id FROM orders GROUP BY order_id, customer_id |
Optimized query:
SELECT DISTINCT order_id, customer_id FROM orders |
Tip 13: Use the ORDER BY clause to sort query results, instead of using the GROUP BY clause.
Inefficient query:
SELECT * FROM orders GROUP BY customer_id ORDER BY order_date |
Optimized query:
SELECT * FROM orders ORDER BY customer_id, order_date |
Tip 14: Use the CAST and CONVERT functions to convert data types, instead of using the + or – operators.
Inefficient query:
SELECT CAST(price AS INT) FROM products |
Optimized query:
SELECT CONVERT(INT, price) FROM products |
Tip 15: Use the IS NULL and IS NOT NULL operators to check for null values, instead of using the = or != operators.
Inefficient query:
SELECT * FROM customers WHERE last_name != NULL |
Optimized query:
SELECT * FROM customers WHERE last_name IS NOT NULL |
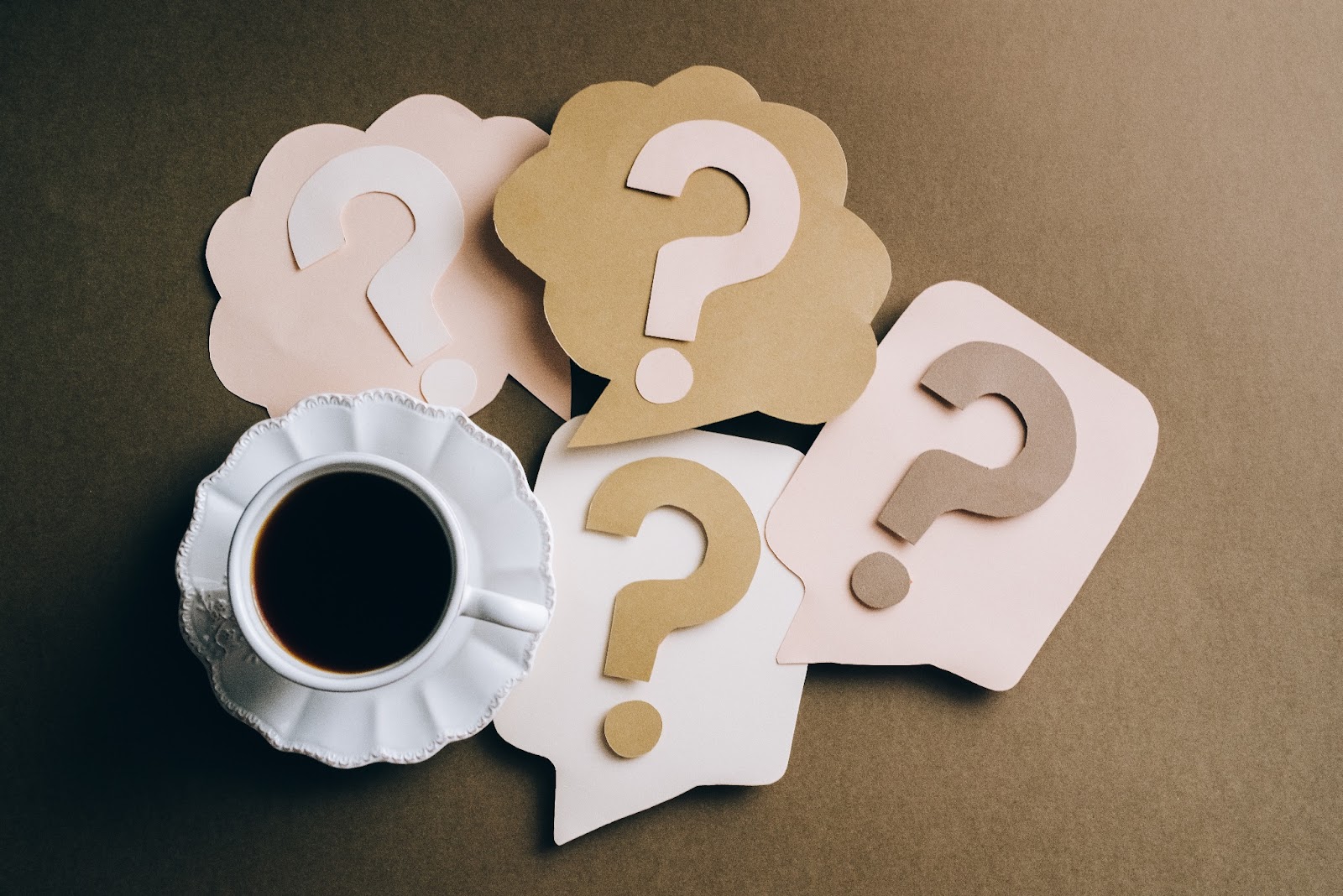
Common FAQs
- Q: What are some of the advanced techniques for optimizing complex queries in MSSQL servers?
A: Some advanced techniques include using indexed views, creating filtered indexes, and using the query optimizer’s query hints.
- Q: How can I identify which queries are causing performance issues in my MSSQL server?
A: You can use tools such as SQL Server Profiler, the SQL Server Management Studio Activity Monitor, and the Dynamic Management Views to identify slow-performing queries.
- Q: What is the role of indexing in complex query optimization in MSSQL servers?
A: Indexing plays a crucial role in query optimization as it allows the query optimizer to quickly locate the data it needs, reducing the number of resources required to execute a query.
- Q: How can I improve the performance of my MSSQL server when dealing with large amounts of data?
A: You can improve performance by partitioning large tables, using the right data types, and using the right indexing strategy.
- Q: How can I optimize my MSSQL server for reporting and analytical workloads?
A: You can optimize your MSSQL server for reporting and analytical workloads by using indexed views, creating columnstore indexes, and using the right data warehousing techniques.
- Q: Can you explain the concept of “parameter sniffing” in MSSQL query optimization?
A: Parameter sniffing is a feature of the MSSQL query optimizer that allows it to optimize a query based onthe specific parameter values used in the execution of the query. This can lead to suboptimal performance if the parameter values used for optimization do not match the typical parameter values used in the query.
- Q: How can I avoid the negative effects of parameter sniffing in MSSQL query optimization?
A: You can avoid the negative effects of parameter sniffing by using the OPTIMIZE FOR query hint, by using local variables instead of parameters, or by using the sp_executesql stored procedure.
- Q: What is the role of statistics in complex query optimization in MSSQL servers?
A: Statistics play a crucial role in query optimization as they provide the query optimizer with information about the distribution of data in a table, which is used to generate an optimal execution plan.
- Q: How can I update statistics on my MSSQL server to improve query optimization?
A: You can update statistics on your MSSQL server by using the UPDATE STATISTICS statement, the sp_updatestats stored procedure, or the SQL Server Management Studio wizard.
- Q: How can I troubleshoot query optimization issues in MSSQL servers?
A: You can troubleshoot query optimization issues by using tools such as the SQL Server Profiler, the SQL Server Management Studio Activity Monitor, and the Dynamic Management Views, as well as by reviewing the execution plan of a query and analyzing the statistics of the tables involved.
Conclusion:
Query optimization is a crucial aspect of maintaining a stable and performant MSSQL server. By implementing the advanced techniques and best practices outlined in this post, SQL Server DBAs and SQL Developers can improve the performance of their queries and ultimately the overall performance of their MSSQL server. Remember, it’s important to always analyze the execution plan of a query, use proper indexing, avoid using the “SELECT *” statement, and use the appropriate clauses, functions and operators for your specific needs. With these tips, you can ensure that your MSSQL server is running at optimal performance.